Zustand: A lightweight state manager for React
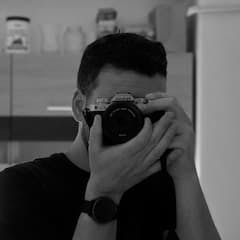
by Petr Rajtslegr
October 13, 2023
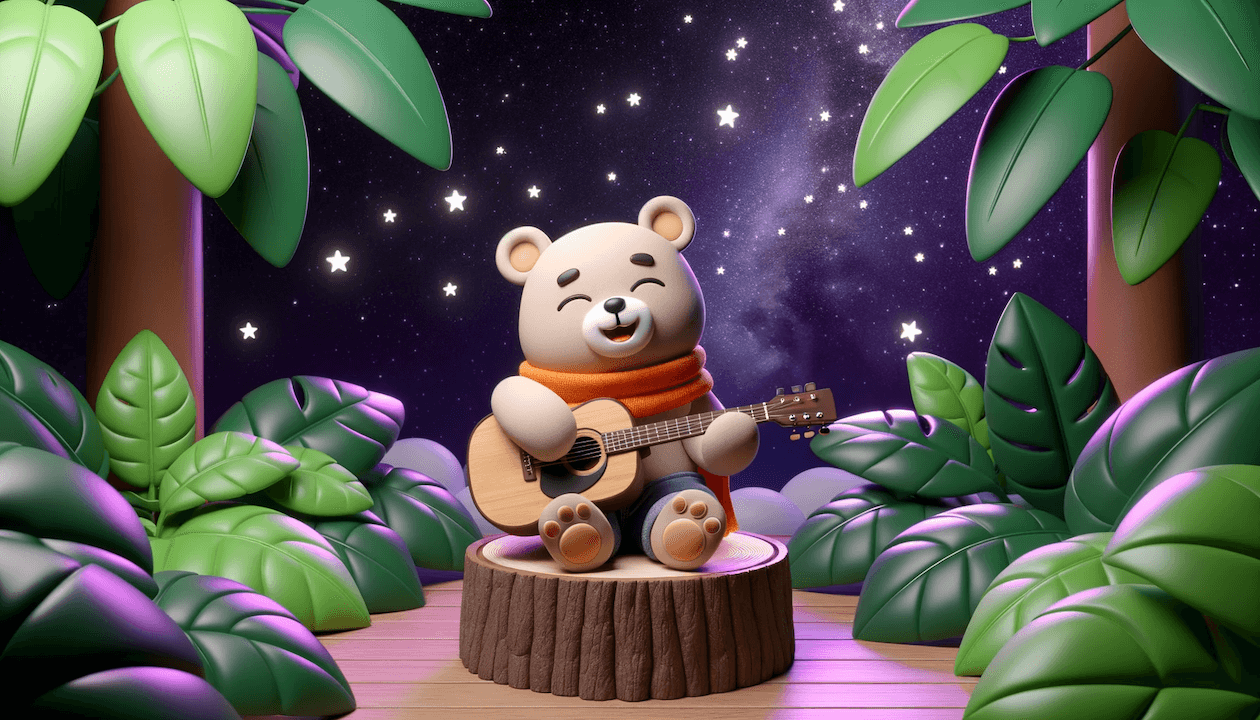
Zustand is a minimalist state management library for React. It offers a simple and intuitive API that helps developers manage global state without the overhead of more complex libraries.
Features:
- Minimal API
- No reducers, actions, or middlewares
- Directly mutable state
- TypeScript support
Setting Up Zustand
First, install the package:
npm install zustand
Creating a Store
With Zustand, creating a store is straightforward:
import { create } from 'zustand';
interface Store {
count: number;
increment: () => void;
};
const useStore = create<Store>((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
}));
export default useStore;
Using the Store in Components
To use the store in your React components:
import React from 'react';
import useStore from './useStore';
const Counter = () => {
const { count, increment } = useStore();
return (
<div>
<p>{count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
Conclusion
Zustand offers a lean and efficient way to handle state management in React applications. Its straightforward API and TypeScript support make it an excellent choice for those looking for a lighter alternative to more extensive state management solutions.
Remember to always consider the needs of your project when selecting a state management library!